2022/3/7
*노드 탐색
요소.parentNode: 선택한 요소의 부모 노드
요소.previousSibiling: 이전 형제 요소
요소.nextSibiling: 다음 형제 요소
요소.firstChild: 현재 요소의 첫 번째 자식 노드
요소.lastChild: 현재 요소의 마지막 자식 노드
요소.childNodes: 자식 노드들
요소.children: 자식 노드들
*요소 조회
1. 요소의 컨텐츠 조회
textContent: 문자열을 넣어주면 된다 (사용 추천)
innterText: 렌더링이 된 텍스트 넣어주기 (비표준 문법)
innerHtml: html이 파싱 된 텍스트. (보안이 취약해 비추)
2. 요소의 속성 (attribute) 접근 / 수정
className: 태그의 클래스 속성 (태그에 클래스 속성이 없다면 생성까지 해줌.)
classList: add, remove, item, toggle 등의 메서드도 제공 (IE10 이상부터 제공)
id: 태그의 id 속성
(attr)은 속성 값
boolean hasAttribute(attr): 메서드임. 속성 지정 여부 확인
String getAttribute(attr): 해당 속성 값 가져오기. 겟 메서드
리턴 타입 x setAttribute(attr, value): attr 속성에 value 값으로 속성 세팅
리턴 타입 x removeAttribute(attr): attr속성 삭제
<body>
<h2 id="test" class ="hehe hoho">hello js</h2>
<script>
let elem = document.getElementById("test");
//ID 통해서 요소를 가져와 변수에 담아주기
console.log(elem, 1);
elem.textContent="helloWorld"; //웹페이지에 출력되는 문자열 바꾸기
console.log(elem.className,2); //클래스 속성값 꺼내기
elem.className="hello"; //클래스 속성값 수정 //클래스 값이 여러개면 갯수 상관없이 무조건 덮어쓰기 해버림. (hehe hoho -> hello)
console.log(elem.className, 3);
console.log(elem.id,4);
elem.id="js";
console.log(elem.id,5);
console.log(elem.hasAttribute("class"),6); //클래스 속성을 가지고 있니..
console.log(elem.hasAttribute("style"),7);
console.log(elem.getAttribute("id"),8); //id 값 가져오기
elem.setAttribute("class", "sunny day"); //이렇게하면 여러개 대체 가능
console.log(elem.getAttribute("class"),9);
elem.removeAttribute("id");
console.log(elem.getAttribute("id"),10); //삭제했기 때문에 null
</script>
</body>
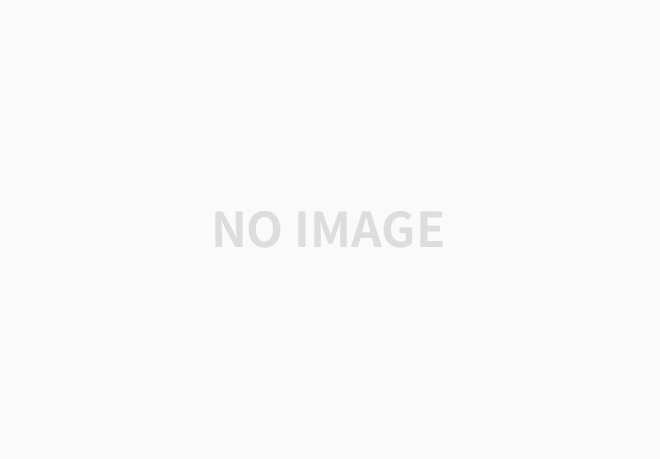
*DOM 조작 (요소 추가)
1. 추가하기를 원하는 노드를 생성한다: createElement(tagName)
2. 텍스트 노드 생성: createTextNode(text) (생략도 가능, 생략하면 콘텐츠가 비어있는 요소)
3. 생성된 요소를 DOM에 추가: appendChild(node) 위에서 만든 노드 매개변수로. (삭제는 removeChild(node))
insertBefore(추가할 요소, 위치)
<body>
<!-- DOM 조작 (요소 추가) -->
<ul class="ultag"></ul>
<script>
// DOM 조작 (요소 추가)
const li =document.createElement("li");//요소 생성
const text = document.createTextNode("javascript"); //텍스트 노드 생성
li.appendChild(text); //텍스트 노드를 생성한 요소에 연결
const ul = document.querySelector("ul.ultag"); //클래스가 있다면 선택자.클래스가 아니라 아이디였으면 ul#ultag
ul.appendChild(li);
//여러개 만드려면
const ul2 = document.querySelector("ul.ultag");
const texts= ["js","jquery","ajax","node.js","react"];
for(var i =0; i<5;i++){
const li =document.createElement("li"); //요소 생성
const text2 = document.createTextNode(texts[i]); //텍스트 노드 생성
li.appendChild(text2); //텍스트 노드를 생성한 요소에 연결
ul2.appendChild(li);//ul 안에 li 태그 추가
}
// insertBefore(추가할 요소, 위치)
const li2 =document.createElement("li"); //요소 생성
const text3 = document.createTextNode("li2"); //텍스트 노드 생성
li2.appendChild(text3); //텍스트 노드를 생성한 요소에 연걸
ul2.insertBefore(li2,ul2.childNodes[0]); //append하면 뒤에 붙는데 insertbefore하고 위치 설정해주면 거기에 들어감
ul.removeChild(document.getElementsByTagName('li')[0]);
</script>
</body>
*classList 메서드
classList.메서드 명()
add(“클래스 속성 값”): class 속성 값 추가
remove(“클래스 속성 값”): class 속성 값 삭제
toggle(“클래스 속성 값”, 조건): 조건은 옵션, class 속성 값 토글 (있으면 없게, 없으면 있게)
item(idx): idx를 이용해 클래스 값 꺼내기
replace(“oldVal”,”newVal”): 존재하는 이전 클래스를 새 클래스로 교체
contains(“클래스 속성 값”): 클래스 속성 값이 존재하는지 존재 여부를 알려준다
#style
요소.style.css 속성 = “값” //보통은 css파일에서 따로 스타일을 다 만들고 이걸 사용하는 건 특별한 경우
<body>
<div>hahaha</div>
<script>
const div = document.querySelector("div");
console.log(div);
//classList
div.className = "hello"; //스크립트로 클래스 속성 추가
div.classList.remove("hello"); //클래스 속성값 삭제
div.classList.add("qwerty");
div.classList.toggle("asdfg"); //클래스 속성이 두개가 됨. asdfg가 없었기 때문에.
div.classList.toggle("asdfg"); //위에선 없어서 추가가 되고 밑에선 있어서 삭제
let i =10;
div.classList.toggle("asdfg", i> 10); //i가 10보다 크면 토글을 해라.
console.log(div.classList.item(0));
div.classList.replace("qwerty", "blackberry");
console.log(div.classList.contains("qwerty"));
//style
div.style.color="blue"; //inline으로 스타일 작성해주는 것과 동일 <div style="color"></div>와 동일
div.style.fontSize="3rem";
</script>
</body>
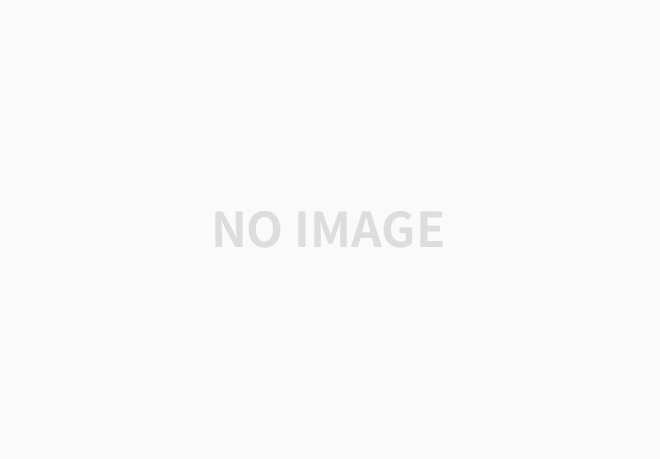
객체 정의 (사용자 정의 객체)
자바 스크립트에서는 객체를 정의할 때 객체 같은 경우는 기본 타입 빼고는 전부 다 클래스 타입이라고 보면 된다. 모든 게 객체다. 원시 타입 제외한 모든 값이 개체다.
: 객체는 객체 리터럴과 생성자 함수 두 가지 방법으로 생성할 수 있다.
1. 객체 리터럴
객체: 이름과 값을 한쌍으로 묶은 데이터들을 여러 개 모은 것.
연관 배열 또는 사전이라고도 부른다.
#객체 생성 (배열은 대괄호 객체는 중괄호)
var 참조 변수 = {}; //빈 객체 생성
var card1 = {shape: “하트”, number: “A”}; //shape변수에 하트가 들어가고 number 변수에 A가 들어간다.
var card2 = {“shape”: “하트”, “number”: “A”};
*프로퍼티: 객체 안의 변수를 속성(property)라 한다. 객체 포함된 데이터 하나 (이름과 값의 쌍)을 가리킴 -> shape: “하트” shpae가 프로퍼티 이름 하트가 프로퍼티 값
*프로퍼티 값을 읽거나 수정할 때는 점(.) 연산자 또는 대괄호([]) 연산자 사용
card1.shape: “하트” 가 나온다. 쌍따옴표 없는 식별자라서 이렇게만 되고
card2[“shape”]: card2는 프로터피 값을 문자열로 넣어줬기 때문에 둘 다 이렇게 가능. 근데 card2 방식은 손이 더 가기 때문에 많이 사용하지 않는다.
card1.color -> undefined. 없는 값이라서.
#프로퍼티 추가, 삭제
추가: 없는 프로퍼티 이름에 값 대입
삭제: delete 연산자 사용
#in 연산자: 프로퍼티 존재 여부 확인
“프로퍼티 명” in 참조 변수 //이런 이름의 프로퍼티가 참조 변수 객체 안에 있니?
#객체 메서드
함수를 객체의 프로퍼티로 담고 있을 때 메서드라고도 표현.
객체 밖에 만든 == 전역/지역 함수
객체 안에 만든 == 메서드
var 참조 변수 = {
프로퍼티 명: 값; //일반 데이터
프로퍼티 명: [], //배열 객체가 값이 될 수도 있고
프로퍼티 명: new Array() //객체 형태로도 넣을 수 있다
프로퍼티 명: function(필요하면 매개변수){
코드 작성. this. 사용할 수 있다. 참조 변수 가리키는 것.
} //익명 함수처럼 넣으면 된다. 이 함수를 쓰고 싶으면 프로퍼티 명 부르면 된다.
};
참조변수 const 써도 안에 값들 수정할 수 있다. 프로퍼티 명은 불가.
<script>
// #객체 생성
//리터럴 방식
const person = {
age: 10,
name: "피카츄",
obj: { //객체 안에 객체 생성 또 가능
a:10,
b:[1,2,3,4],
c: function(){}
},
//객체 메서드
getInfo: function(){
console.log(this.age); //메서드 안에서 내부 요소를 쓰고 싶을때는 this를 붙여야 한다.
console.log(this.name);
console.log("hello");
}
}
person.getInfo();
person.obj.a=200;
let abc = {};
abc.test = "hello";
abc.func = function(){
}
console.log(person); //전체 접근
console.log(person.age); //하나씩 접근
console.log(person.name);
person.mobile = "010-1111-2222"; //프로퍼티 추가
console.log(person);
delete person.mobile; //프로퍼티 삭제
console.log(person);
console.log("test" in person); //f
console.log("age" in person); //t
console.log("toString" in person); //t. 오브젝트라는 최고 부모를 모두 상속 받음 그래서 이 객체를 보면 프로토타입이 오브젝트 되어있어. 부모 클래스는 오브젝트. 부모가 물려준 기능들 중에 투스트링이 있다. 오브젝트가 물려준 투스트링이 person 안에 있기 때문에 true.
</script>
2. 객체 생성자로 정의
#1. 생성자 정의
function 생성자 명(필요하면 매개변수){
//코드}
객체인지 일반 함수인지 구분하기 위해서 생성자명은 첫 글자 대문자로 정의. 일반 함수는 소문자. 객체로 쓸 거면 첫 글자 대문자.
//객체 리터럴 (간단하게 데이터를 객체로 묶어서 당장 써야 할 때)
var card1 = {shape: “하트”, number: “A”};
//객체 생성자 (다양한 기능을 넣고 여기저기서 많이 써야 하면 이 방법)
function Card(shape, number){
this.shape = shape;
this.numner = number;
}
//객체 생성자 호출
var card = new Card(“하트”, “A”);
card.shape -> “하트”
card.number -> “A”
card.메서드명();
<script>
//객체 생성자
function Inbody(name, age, mobile, height, weight){
//프로퍼티
this.name = name;
this.age = age;
this.mobile = mobile;
this.height = height;
this.weight = weight;
//메서드
this.getInfo = function(){
let str = "이름: "+ this.name;
str += ", 나이: " + this.age;
str += ", 전화번호: " + this.mobile;
str += ", 키: " + this.height;
str += ", 몸무게: " + this.weight;
return str;
}
this.getBalanceResult=function(){
this.minW = (this.height - 100) * 0.9 -5 ;
this.maxW = (this.weight -100) * 0.9 + 5;
if(this.weight >= this.minW && this.weight <= this.maxW){
return "정상 몸무게";
}else if ( this.weight < this.minW){
return "몸무게 미달";
}else{
return "몸무게 초과";
}
} //getBalanceResult
} //Inbody
var pika = new Inbody("피카츄", 10, "010-1111-2222", 65, 20);
console.log(pika);
console.log(pika.getInfo());
console.log(pika.getBalanceResult());
pika.test = 10;
pika.hello = function() {console.log("hello")};
console.log(pika);
3. class: ES6
클래스기 때문에 상속이 가능하다.
class 클래스 명{
constructor(){ //생성자
this.name = “hello”; //프로퍼티 추가
}
}
//class
class Human{
constructor(){}
}
class Person extends Human{ //클래스로 만들었기 때문에 상속이 가능
constructor(){
super(); //상속 받았으면 부모 생성자 호출해주기
this.age = 10; //생성자 안에서 변수 생성
this.name = "pika";
}
getInfo(){
return "나이: "+ this.age+", 이름: " + this.name;
}
}
let me = new Person();
console.log(me);
console.log(me.getInfo());